This project was inspired by the work of Andy Stanford-Clark (@andyc) and Lucy Rogers (@drlucyrogers). They used NodeRED to control a Maplin Action Dino kit via the Twitter hashtag #wakedino. When a tweet containing #wakedino is found by the NodeRED script, the motor is started, which in turn makes the dinosaur nod up and down.
I opted to recreate their work in Python using the Tweepy library. I also wanted to build upon the NodeRed implementation with the addition of sound, and the capturing and tweeting of an image.
Powering the Dinosaur
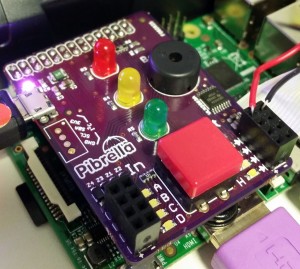
Pibrella
The first problem to solve was how to control the motor. Connecting a motor directly to the GPIO pins is bad; at best your Pi will have a drop in power and restart, (aka “brown out”; a bit like when your lights at home dim briefly) there is also the potential to damage the GPIO. This can happen due to the high power requirements (the amount of amps) to start the motor, there is also a risk of a surge of power when the motor stops.
An Open Collect Driver circuit; like the one shown in Lucy’s blog post at Element14, or an H-bridge motor driver would do the trick. However I had neat and tidy solution in my “toy” box; the ever versatile Pibrella from Cyntech & Pimoroni. It has an ULN2003 on board (a chip containing 8 darlington pairs or transistors) which could do the heavy lifting for the motor. It also has a button, the use of which will become apparent later, and LEDs for some status indication.
Rocking Dino
With the motor wired to the E connections (as shown in the picture), controlling the motor is as as easy as…
1 2 3 4 5 6 7 8 9 | import pibrella #turn on pibrella.output.e.on() #do stuff, maybe time.sleep(x) #turn off pibrella.output.e.off() |
Except…. the motor is geared for 1.5v operation and the Pibrella outputs 5v on these sockets. Needless to say my poor dinosaur nearly sued me for whiplash as it bobbed up and down at a fantastic speed!
To solve the problem I used Pulse Width Modulation (PWM)
1 2 3 4 5 6 7 8 9 | import pibrella #turn on pibrella.output.e.pwm(50,12) #do stuff, maybe time.sleep(x) #turn off pibrella.output.e.off() |
The value 12 is the key bit here; this is the duty cycle. The duty cycle is the amount of time the motor is on, per cycle, as a percentage. The frequency (the number of cycles/second) is set to 50Hz; (now for the maths)
50Hz = 1000 milliseconds / 50 = 20 ms, so every cycle is 20 ms
12% of 20 ms is 2.4 ms, so the motor is on for 2.4 ms and off for 17.6 ms
or another way of putting it….. after trial and error, a duty cycle of 12 made the dino slow down to about the same speed as when wired to the 1.5v battery.
Reacting to Twitter
The original work used the built in Twitter module of NodeRED to search for new tweets. As this is all done in Python I used the Tweepy library. To save reinventing the wheel I suggest you go to Alex Eames’s (@RasPiTV) site and use the same guide as me for getting Tweepy up and running. Make sure you follow all the instructions as the default Tweepy install is not all it’s cracked up to be!
The Python code searches for new tweets containing #camjam, #eghamjam or #wakedino. When a matching tweet is found, the motor is activated via the Pibrella, an LED flashes, and a random dinosaur sound effect is played.
http://www.dinosaurfact.net/Sounds.php
Tweeting Dino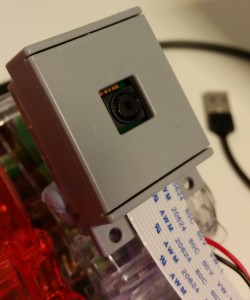
Now for the Pibrellas “Big Red Button”. By adding the Raspberry Pi camera module my dinosaur can now tell the world who’s pushed the button!
Once the button has been pressed, a preview window for the camera is displayed on screen. After 5 seconds a snapshot is taken and then sent to Twitter. See the @douthinkhesaur Twitter account for the “puny humans” my dinosaur has captured so far.
To do all this I’m using the Tweepy library again, this time in conjunction with the PiCamera library. The latter allows me a lot more control over the workings of the camera and its output.
Further Info
Source: https://github.com/ForToffee/DinoTweet
A lot of the Twitter code is based on examples provided by Alex Eames.
Twyton is another Twitter library you could use